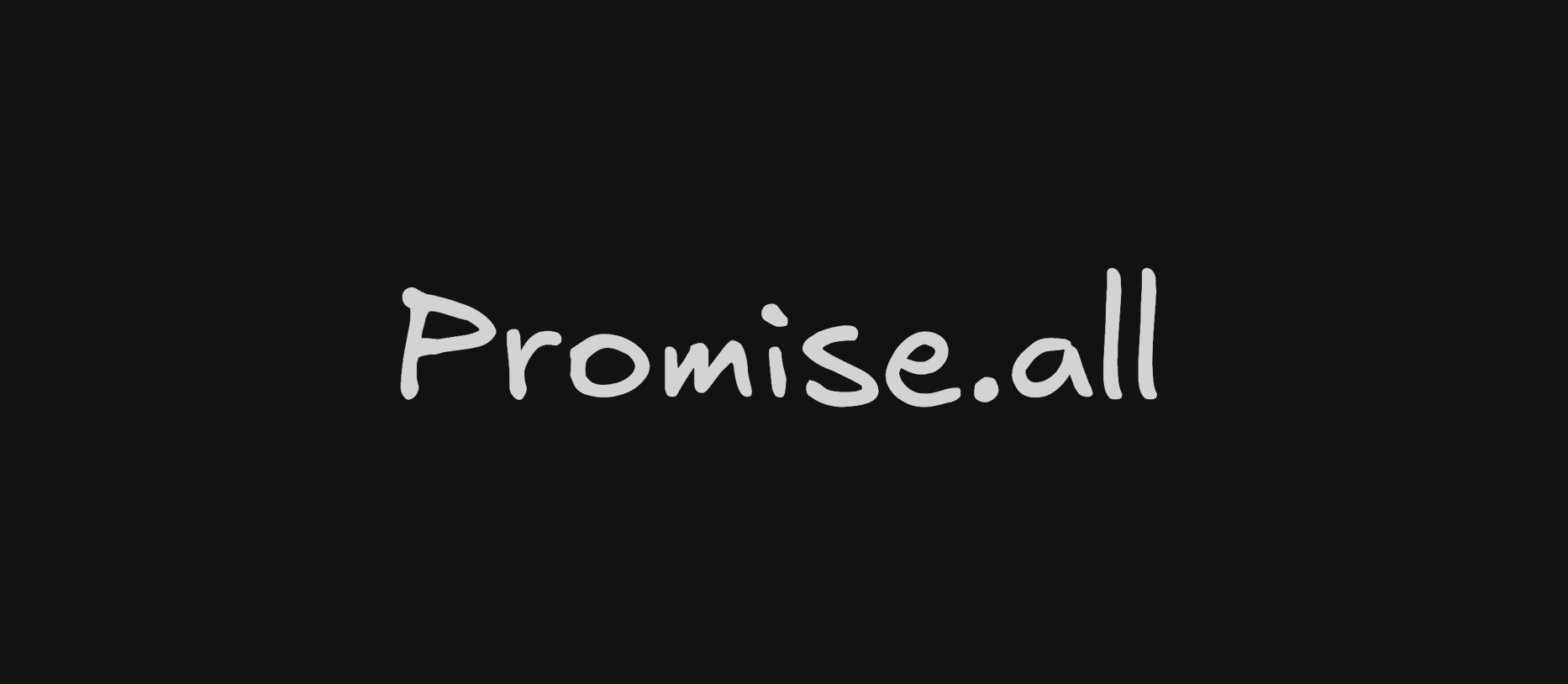
Build Promise.all() From Scratch
Published at January 12, 2025 by Aulia Rahman
Last week I got curious about how Promise.all() works behind the scenes. Instead of just reading the docs, I decided to build it from scratch.
The Challenge
The core idea is simple - it takes an array of promises and waits for all of them to resolve. But how do you actually track multiple promises at once while maintaining their order?
My First Attempt
I started with what I knew - a new Promise that would wrap everything:
I needed to track results and know when everything was finished. After some thought, I figured I'd need:
- An array to store results
- A way to count unfinished promises
The Breakthrough
Each promise needed to:
- Store its result in the right spot
- Tell us when it's done
- Not mess up if other promises finish first
Here's what I came up with:
The unresolved
counter was key here. When it hits zero, we know every promise has finished. And using forEach
with the index let me keep everything in order.
The Edge Cases
I had to handle a few things:
- Empty arrays
- Immediate rejection if any promise fails
- Maintaining the original order
Testing It Out
Here's a quick test:
It worked! Even with promises that resolved at different times, everything came back in order.
What I Learned
Building Promise.all() helped me understand:
- The mechanics behind promise handling
- How to track multiple async operations
- Sometimes a simple counter is all you need
Next time you use Promise.all(), you'll know there's just a counter and array making it all work.